15.1 API Conventions¶
15.1.1 Function arguments¶
Naming Convention
In the definition of the MOSEK Optimizer API for Python a consistent naming convention has been used. This implies that whenever for example numcon
is an argument in a function definition it indicates the number of constraints. In Table 15.1 the variable names used to specify the problem parameters are listed.
API name |
API type |
Dimension |
Related problem parameter |
---|---|---|---|
|
|
||
|
|
||
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
The relation between the variable names and the problem parameters is as follows:
The quadratic terms in the objective:
The linear terms in the objective :
The fixed term in the objective :
The quadratic terms in the constraints:
The linear terms in the constraints:
Information about input/output arguments
The following are purely informational tags which indicate how MOSEK treats a specific function argument.
(input) An input argument. It is used to input data to MOSEK.
(output) An output argument. It can be a user-preallocated data structure, a reference, a string buffer etc. where MOSEK will output some data.
(input/output) An input/output argument. MOSEK will read the data and overwrite it with new/updated information.
15.1.2 Bounds¶
The bounds on the constraints and variables are specified using the variables bkc
, blc
, and buc
. The components of the integer array bkc
specify the bound type according to Table 15.2
Symbolic constant |
Lower bound |
Upper bound |
---|---|---|
finite |
identical to the lower bound |
|
minus infinity |
plus infinity |
|
finite |
plus infinity |
|
finite |
finite |
|
minus infinity |
finite |
For instance bkc[2]=
boundkey.lo
means that
Finally, the numerical values of the bounds are given by
The bounds on the variables are specified using the variables bkx
, blx
, and bux
in the same way. The numerical values for the lower bounds on the variables are given by
15.1.3 Vector Formats¶
Three different vector formats are used in the MOSEK API:
Full (dense) vector
This is simply an array where the first element corresponds to the first item, the second element to the second item etc. For example to get the linear coefficients of the objective in task
with numvar
variables, one would write
c = zeros(numvar,float)
task.getc(c)
Vector slice
A vector slice is a range of values from first
up to and not including last
entry in the vector, i.e. for the set of indices i
such that first <= i < last
. For example, to get the bounds associated with constrains 2 through 9 (both inclusive) one would write
upper_bound = zeros(8,float)
lower_bound = zeros(8,float)
bound_key = array([0] * 8)
task.getconboundslice(2, 10,
bound_key,lower_bound,upper_bound)
Sparse vector
A sparse vector is given as an array of indexes and an array of values. The indexes need not be ordered. For example, to input a set of bounds associated with constraints number 1, 6, 3, and 9, one might write
bound_index = [ 1, 6, 3, 9]
bound_key = [boundkey.fr,boundkey.lo,boundkey.up,boundkey.fx]
lower_bound = [ 0.0, -10.0, 0.0, 5.0]
upper_bound = [ 0.0, 0.0, 6.0, 5.0]
task.putconboundlist(bound_index,
bound_key,lower_bound,upper_bound)
15.1.4 Matrix Formats¶
The coefficient matrices in a problem are inputted and extracted in a sparse format. That means only the nonzero entries are listed.
15.1.4.1 Unordered Triplets¶
In unordered triplet format each entry is defined as a row index, a column index and a coefficient. For example, to input the
subi = [ 1, 3, 5 ]
subj = [ 2, 3, 4 ]
cof = [ 1.1, 4.3, 0.2 ]
task.putaijlist(subi,subj,cof)
Please note that in some cases (like Task.putaijlist
) only the specified indexes are modified — all other are unchanged. In other cases (such as Task.putqconk
) the triplet format is used to modify all entries — entries that are not specified are set to
15.1.4.2 Column or Row Ordered Sparse Matrix¶
In a sparse matrix format only the non-zero entries of the matrix are stored. MOSEK uses a sparse packed matrix format ordered either by columns or rows. Here we describe the column-wise format. The row-wise format is based on the same principle.
Column ordered sparse format
A sparse matrix in column ordered format is essentially a list of all non-zero entries read column by column from left to right and from top to bottom within each column. The exact representation uses four arrays:
asub
: Array of size equal to the number of nonzeros. List of row indexes.aval
: Array of size equal to the number of nonzeros. List of non-zero entries of ordered by columns.ptrb
: Array of sizenumcol
, whereptrb[j]
is the position of the first value/index inaval
/asub
for the -th column.ptre
: Array of sizenumcol
, whereptre[j]
is the position of the last value/index plus one inaval
/asub
for the -th column.
With this representation the values of a matrix numcol
columns are assigned using:
As an example consider the matrix
which can be represented in the column ordered sparse matrix format as
Fig. 15.1 illustrates how the matrix
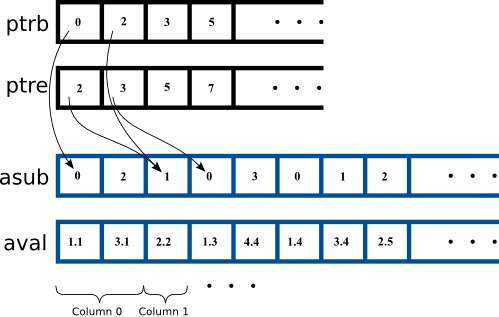
Fig. 15.1 The matrix
Column ordered sparse format with nonzeros
Note that nzc[j] := ptre[j]-ptrb[j]
is exactly the number of nonzero elements in the asub
, aval
, ptrb
, nzc
. The matrix
Row ordered sparse matrix
The matrix