11.6 Inner and outer Löwner-John Ellipsoids¶
In this section we show how to compute the Löwner-John inner and outer ellipsoidal approximations of a polytope. They are defined as, respectively, the largest volume ellipsoid contained inside the polytope and the smallest volume ellipsoid containing the polytope, as seen in Fig. 11.7.
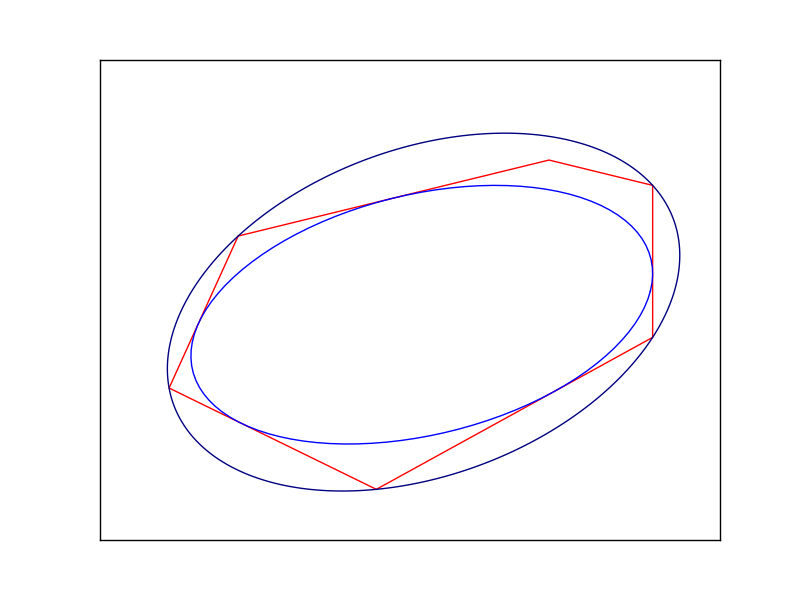
Fig. 11.7 The inner and outer Löwner-John ellipse of a polygon.¶
For further mathematical details, such as uniqueness of the two ellipsoids, consult [BenTalN01]. Our solution is a mix of conic and semidefinite programming. Among other things, in Sec. 11.6.3 (Bound on the Determinant Root) we show how to implement bounds involving the determinant of a PSD matrix.
11.6.1 Inner Löwner-John Ellipsoids¶
Suppose we have a polytope given by an h-representation
and we wish to find the inscribed ellipsoid with maximal volume. It will be convenient to parametrize the ellipsoid as an affine transformation of the standard disk:
Every non-degenerate ellipsoid has a parametrization such that \(C\) is a positive definite symmetric \(n\times n\) matrix. Now the volume of \(\mathcal{E}\) is proportional to \(\mbox{det}(C)^{1/n}\). The condition \(\mathcal{E}\subseteq\mathcal{P}\) is equivalent to the inequality \(A(Cu+d)\leq b\) for all \(u\) with \(\|u\|_2\leq 1\). After a short computation we obtain the formulation:
where \(X_i\) denotes the \(i\)-th row of the matrix \(X\). This can easily be implemented using Fusion, where the sequence of conic inequalities can be realized at once by feeding in the matrices \(b-Ad\) and \(AC\).
public static Tuple<double[], double[]> lownerjohn_inner(double[][] A, double[] b)
{
using( Model M = new Model("lownerjohn_inner"))
{
int m = A.Length;
int n = A[0].Length;
// Setup variables
Variable t = M.Variable("t", 1, Domain.GreaterThan(0.0));
Variable C = det_rootn(M, t, n);
Variable d = M.Variable("d", n, Domain.Unbounded());
// (bi - ai^T*d, C*ai) \in Q
for (int i = 0; i < m; ++i)
M.Constraint("qc" + i, Expr.Vstack(Expr.Sub(b[i], Expr.Dot(A[i], d)), Expr.Mul(C, A[i])),
Domain.InQCone().Axis(0) );
// Objective: Maximize t
M.Objective(ObjectiveSense.Maximize, t);
M.Solve();
return Tuple.Create(C.Level(), d.Level());
}
}
The only black box is the method det_rootn
which implements the constraint \(t\leq \mbox{det}(C)^{1/n}\). It will be described in Sec. 11.6.3 (Bound on the Determinant Root).
11.6.2 Outer Löwner-John Ellipsoids¶
To compute the outer ellipsoidal approximation to a polytope, let us now start with a v-representation
of the polytope as a convex hull of a set of points. We are looking for an ellipsoid given by a quadratic inequality
whose volume is proportional to \(\mbox{det}(P)^{-1/n}\), so we are after maximizing \(\mbox{det}(P)^{1/n}\). Again, there is always such a representation with a symmetric, positive definite matrix \(P\). The inclusion conditions \(x_i\in\mathcal{E}\) translate into a straightforward problem formulation:
and then directly into Fusion code:
public static Tuple<double[], double[]> lownerjohn_outer(double[,] x)
{
using( Model M = new Model("lownerjohn_outer") )
{
int m = x.GetLength(0);
int n = x.GetLength(1);
// Setup variables
Variable t = M.Variable("t", 1, Domain.GreaterThan(0.0));
Variable P = det_rootn(M, t, n);
Variable c = M.Variable("c", Domain.Unbounded().WithShape(1,n));
// (1, P(*xi+c)) \in Q
M.Constraint("qc",
Expr.Hstack(Expr.Ones(m), Expr.Sub(Expr.Mul(x,P), Expr.Repeat(c,m,0))),
Domain.InQCone().Axis(1));
// Objective: Maximize t
M.Objective(ObjectiveSense.Maximize, t);
M.Solve();
return Tuple.Create(P.Level(), c.Level());
}
}
11.6.3 Bound on the Determinant Root¶
It remains to show how to express the bounds on \(\mbox{det}(X)^{1/n}\) for a symmetric positive definite \(n\times n\) matrix \(X\) using PSD and conic quadratic variables. We want to model the set
A standard approach when working with the determinant of a PSD matrix is to consider a semidefinite cone
where \(Z\) is a matrix of additional variables and where we intuitively identify \(\mbox{Diag}(Z)=\{\lambda_1,\ldots,\lambda_n\}\) with the eigenvalues of \(X\). With this in mind, we are left with expressing the constraint
but this is exactly the geometric mean cone Domain.InPGeoMeanCone
. We obtain the following model:
public static Variable det_rootn(Model M, Variable t, int n)
{
// Setup variables
Variable Y = M.Variable(Domain.InPSDCone(2 * n));
Variable X = Y.Slice(new int[]{0, 0}, new int[]{n, n});
Variable Z = Y.Slice(new int[]{0, n}, new int[]{n, 2 * n});
Variable DZ = Y.Slice(new int[]{n, n}, new int[]{2 * n, 2 * n});
// Z is lower-triangular
int[,] low_tri = new int[n*(n-1)/2,2];
int k = 0;
for(int i = 0; i < n; i++)
for(int j = i+1; j < n; j++)
{ low_tri[k,0] = i; low_tri[k,1] = j; ++k; }
M.Constraint(Z.Pick(low_tri), Domain.EqualsTo(0.0));
// DZ = Diag(Z)
M.Constraint(Expr.Sub(DZ, Expr.MulElm(Z, Matrix.Eye(n))), Domain.EqualsTo(0.0));
// t^n <= (Z11*Z22*...*Znn)
M.Constraint(Expr.Vstack(DZ.Diag(), t), Domain.InPGeoMeanCone());
// Return an n x n PSD variable which satisfies t <= det(X)^(1/n)
return X;
}